Java is one of the most popular and versatile programming languages, widely used in various applications, from mobile apps to large-scale enterprise systems. If you're new to Java or programming in general, this basic Java tutorial for beginners is the perfect starting point. We'll cover essential concepts, walk you through coding examples, and guide you on how to build a strong foundation in Java.
What You Will Learn:
Introduction to Java and its features
Setting up your Java development environment
Basic Java syntax and concepts
Object-oriented programming (OOP) in Java
Moving towards advanced Java programming concepts
Resources and tips to further enhance your learning
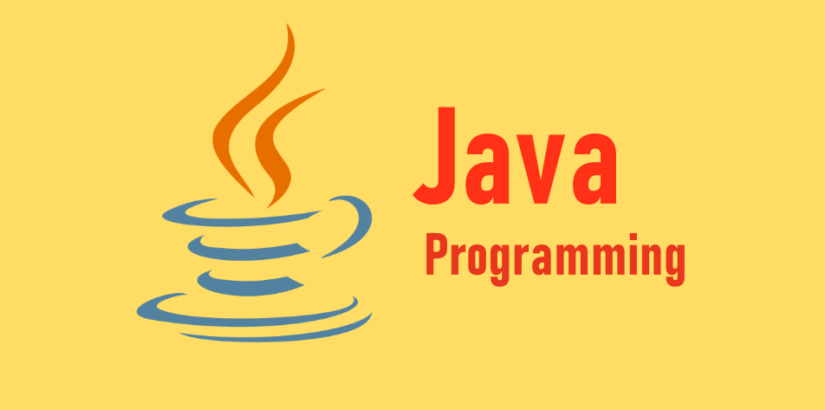
Introduction to Java
Java is a high-level, object-oriented programming language known for its platform independence, meaning that Java code can run on any device that has the Java Virtual Machine (JVM). It’s commonly used for building Android apps, web applications, and server-side software. Java's "write once, run anywhere" principle makes it a favorite among developers.
Setting Up Your Java Development Environment
1. Install Java Development Kit (JDK)
Download and install the latest version of JDK from the official Oracle website.
2. Choose an Integrated Development Environment (IDE)
An IDE simplifies the coding process. Popular choices include Eclipse, IntelliJ IDEA, and NetBeans.
3. Configure Your IDE
Set up your IDE to recognize the JDK you've installed, allowing you to compile and run Java programs.
Basic Java Syntax and Concepts
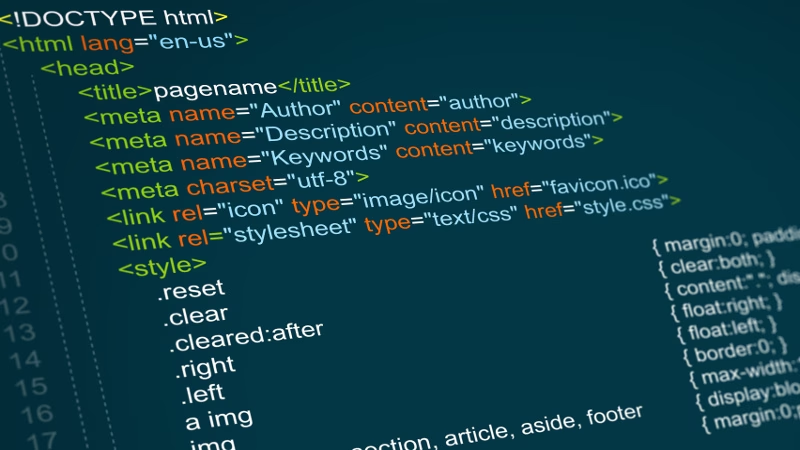
1. Hello World Program
Every programming tutorial starts with a simple "Hello World" program. In Java, it looks like this:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
- public class HelloWorld
declares a class named HelloWorld.
- public static void main(String[] args)
is the entry point of any Java application.
- System.out.println("Hello, World!");
prints the message to the console.
2. Variables and Data Types
Variables in Java store data values. You must declare a variable with a data type before using it:
int number = 10;
double pi = 3.14;
char letter = 'A';
String message = "Welcome to Java!";
- Primitive Data Types: int, double, char, boolean, etc.
- Non-Primitive Data Types: String, arrays, classes, etc.
3. Operators
Java supports various operators for arithmetic, comparison, logical operations, and more:
int a = 5;
int b = 10;
int sum = a + b; // Arithmetic operator
boolean isEqual = (a == b); // Comparison operator
4. Control Flow Statements
Control flow statements like if
, else
, switch
, for
, while
, and do-while
allow you to control the flow of your program:
if (a > b) {
System.out.println("a is greater than b");
} else {
System.out.println("b is greater than or equal to a");
}
for (int i = 0; i < 5; i++) {
System.out.println("Iteration " + i);
}
Object-Oriented Programming (OOP) in Java
1. Classes and Objects
A class is a blueprint for creating objects. An object is an instance of a class:
public class Car {
String model;
int year;
public Car(String model, int year) {
this.model = model;
this.year = year;
}
public void displayInfo() {
System.out.println("Model: " + model + ", Year: " + year);
}
}
public class Main {
public static void main(String[] args) {
Car myCar = new Car("Toyota", 2020);
myCar.displayInfo();
}
}
- Car
is a class with properties (model, year) and methods (displayInfo).
- myCar
is an object created from the Car
class.
2. Inheritance
Inheritance allows a new class to inherit properties and behaviors from an existing class:
public class ElectricCar extends Car {
int batteryLife;
public ElectricCar(String model, int year, int batteryLife) {
super(model, year);
this.batteryLife = batteryLife;
}
public void displayBatteryLife() {
System.out.println("Battery Life: " + batteryLife + " hours");
}
}
- ElectricCar
inherits from Car
using the extends
keyword.
Moving Towards Advanced Java Programming Concepts
Once you are comfortable with the basics, you can explore advanced Java programming concepts like:
Java Collections Framework: Understanding ArrayList, HashMap, HashSet, etc., for data storage and manipulation.
Exception Handling: Learn how to handle errors using try, catch, finally, and custom exceptions.
Java I/O: Reading and writing data using input/output streams.
Multithreading: Learn how to execute multiple threads simultaneously for efficient resource utilization.
For an in-depth understanding of these concepts, you can refer to the best Java tutorial for beginners on YouTube that cover advanced topics in a simplified manner.
Tips for Learning Java Effectively
Practice Regularly: The key to mastering Java is consistent practice. Try coding small projects to apply what you've learned.
Join Java Communities: Participate in forums like Stack Overflow and JavaRanch to seek help and advice.
Follow Tutorials and Courses: Online courses on platforms like Coursera, Udemy, and YouTube offer comprehensive tutorials, including basic and advanced Java programming concepts.
Conclusion
Learning Java is an excellent choice for aspiring programmers. This basic Java tutorial for beginners has covered fundamental concepts, setting up your environment, and the basics of object-oriented programming. By understanding and practicing these core concepts, you'll build a solid foundation that prepares you for advanced Java programming concepts and real-world application development. Remember, consistency and practice are key, so keep coding and exploring more complex topics as you progress on your Java programming journey.