PHP, which stands for Hypertext Preprocessor, is a widely-used server-side scripting language designed for web development but also used as a general-purpose language. It is known for its role in creating dynamic and interactive websites. If you are new to web development or programming in general, this guide on the basics of PHP programming language will help you understand its core concepts and how to start coding effectively.
Introduction to PHP
PHP is embedded within HTML and can be used to create dynamic web pages that interact with databases and perform various functions on the server side. Unlike client-side languages like JavaScript, PHP code is executed on the server, and the result is sent to the client’s browser.
Key Features of PHP:
Server-Side Execution: PHP scripts run on the server and generate HTML which is then sent to the client.
Database Integration: PHP can interact with various databases, such as MySQL, to store and retrieve data.
Flexibility and Compatibility: It works across different platforms and integrates easily with other technologies.
Setting Up a PHP Development Environment
1. Install a Local Server
To run PHP scripts locally, you need a server environment. Popular options include XAMPP and WampServer, which bundle Apache, MySQL, and PHP together.
2. Choose a Code Editor
A code editor or Integrated Development Environment (IDE) is essential for writing PHP code. Popular editors include Visual Studio Code, Sublime Text, and PHPStorm.
3. Configure Your Environment
Install and configure the local server and set up your editor to work with PHP files. Ensure that your PHP environment is correctly set up to run scripts and view output.
Basic Syntax and Concepts in PHP
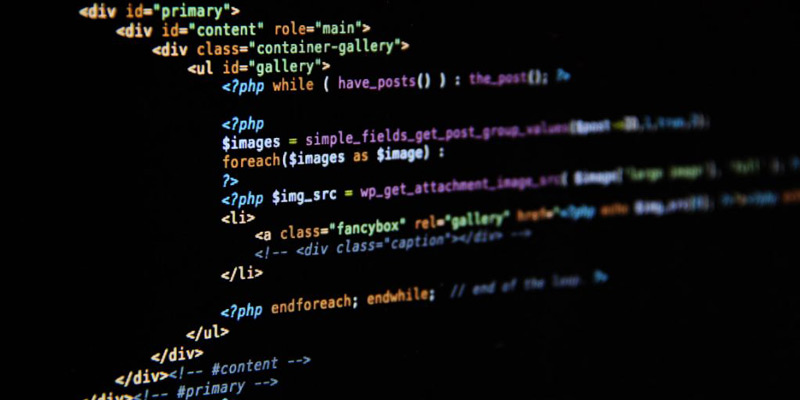
1. Hello World Program
Your first PHP script can be a simple “Hello, World!” example:
<?php
echo "Hello, World!";
?>
- <?php and ?> are PHP tags that open and close PHP code blocks.
- echo is a function used to output text to the web page.
2. Variables and Data Types
Variables in PHP start with a dollar sign $ and do not require explicit declaration of data types:
$number = 10;
$pi = 3.14;
$letter = 'A';
$message = "Welcome to PHP!";
- Primitive Data Types: Integer, float, string, boolean.
- Complex Data Types: Arrays, objects.
3. Operators
PHP supports various operators for arithmetic, comparison, and logical operations:
$a = 5;
$b = 10;
$sum = $a + $b; // Addition
$isEqual = ($a == $b); // Comparison
$logicalAnd = ($a > 0 && $b > 0); // Logical AND
4. Control Flow Statements
Control flow statements allow you to make decisions and repeat actions in your PHP scripts:
if ($a > $b) {
echo "a is greater than b";
} else {
echo "b is greater than or equal to a";
}
for ($i = 0; $i < 5; $i++) {
echo "Iteration " . $i;
}
Core PHP Functions and Examples
1. String Functions
- strlen($string): Returns the length of a string.
- str_replace($search, $replace, $subject): Replaces all occurrences of a substring.
Example:
$name = "PHP Programming";
echo strlen($name); // Output: 16
echo str_replace("Programming", "Basics", $name); // Output: PHP Basics
2. Array Functions
- array_push($array, $value): Adds one or more elements to the end of an array.
- array_merge($array1, $array2): Merges two or more arrays.
Example:
$colors = array("Red", "Green");
array_push($colors, "Blue");
print_r($colors); // Output: Array ( [0] => Red [1] => Green [2] => Blue )
3. Date and Time Functions
- date('Y-m-d'): Returns the current date in the format YYYY-MM-DD.
- strtotime($time): Parses an English textual datetime description into a Unix timestamp.
Example:
echo date('Y-m-d'); // Output: Current date
echo strtotime("next Monday"); // Output: Unix timestamp for next Monday
Moving Towards Advanced PHP Programming Concepts
Once you are comfortable with the basics of PHP programming language, you can explore advanced concepts to enhance your skills.
- Object-Oriented Programming (OOP): Learn about classes, objects, inheritance, and polymorphism.
- Error Handling: Understand how to handle errors and exceptions using try, catch, and finally.
- Database Interaction: Use PHP with MySQL or other databases to perform CRUD (Create, Read, Update, Delete) operations.
For a deeper dive into these topics, look for resources and tutorials that cover the basics of PHP language and the basic concepts of PHP.
Resources for Further Learning
- PHP Manual: The official PHP documentation for in-depth understanding.
- Codecademy PHP Course: Interactive learning for beginners.
- YouTube Tutorials: Visual tutorials for practical coding examples.
Conclusion
Understanding the basics of PHP programming language is the first step towards mastering web development. By familiarizing yourself with PHP syntax, functions, and control structures, you set a strong foundation for more advanced topics. Practice coding regularly and explore additional resources to enhance your basic knowledge of PHP. With dedication and continuous learning, you’ll be able to develop dynamic and interactive web applications using PHP.